Google の Firebase Authentication を使えば、自前のデータベース無しで ユーザー認証できる。React,webpack,Visual Studio Code(VS Code),Vue Router,jquery などに一切依存しないシンプルな実装サンプル。スタティックファイルだけの既存サイトに javascriptだけで 新規登録・ログイン・ログアウトを追加する備忘録。
▼新規登録・ログイン ▲ログアウト
前提は
●以前の「Firebase Functions 1」 で「プロジェクト」と「ウェブ(web appli)」まで作成済。
●firebasejs 10.1.0 (Firebase v10)
...▼
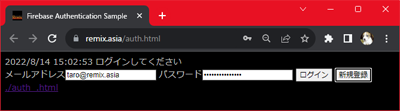
▼新規登録・ログイン ▲ログアウト
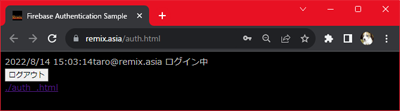
前提は
●以前の「Firebase Functions 1」 で「プロジェクト」と「ウェブ(web appli)」まで作成済。
●firebasejs 10.1.0 (Firebase v10)
...▼
■Firebase Console
https://console.firebase.google.com/
トップメニュー / 構築 / Authentication
▼
▼
Sign-in method
[ネイティブのプロバイダ] メール/パスワード
▼
▼
▼
プロジェクトの概要 / プロジェクトの設定
▼
SDKの設定と構成 / CDN
firebaseconfigパラメータを使用。
▼
auth.html ファイルへフォームと javascript を実装。
https://remix.asia/auth.html
メール と パスワード を入力して 新規登録。
▼
https://remix.asia/auth.html
↓↑遷移してもOK
https://remix.asia/auth_.html
このままでは、auth.htm, auth_.html をダウンロード・コピー出来る為 イタズラされる恐れ。有効な https サイトからに制限する場合...▼
■Google Cloud Console
https://console.cloud.google.com/home/
APIとサービス / 認証情報
▼
■Firebase Console でアカウント管理
https://console.firebase.google.com/
Users
https://console.firebase.google.com/

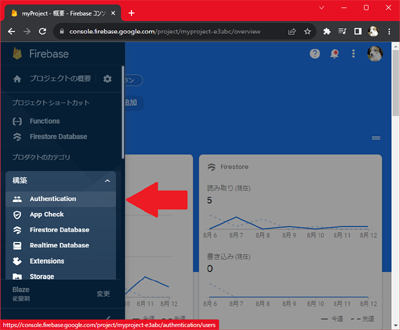
▼
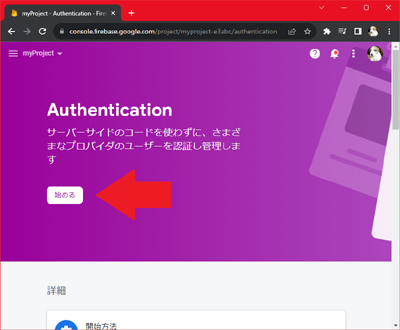
▼
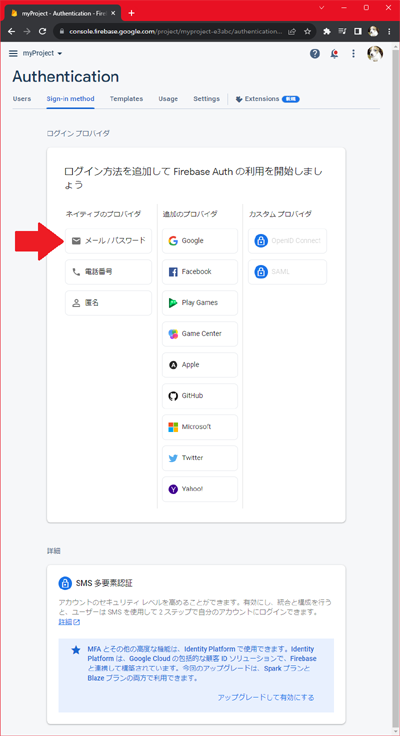
[ネイティブのプロバイダ] メール/パスワード
▼
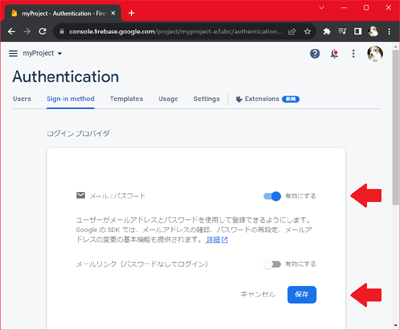
▼
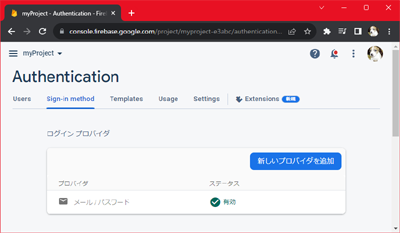
▼
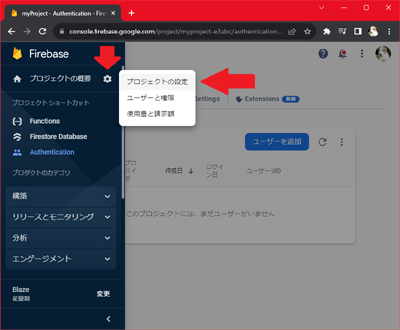
▼
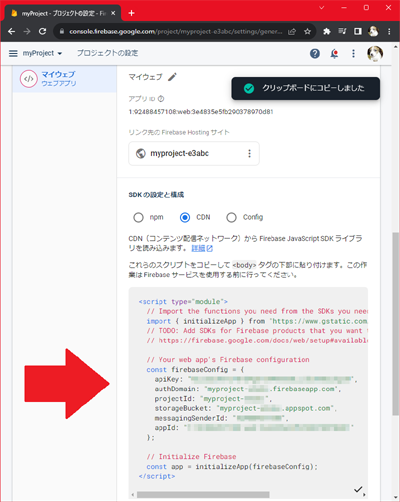
firebaseconfigパラメータを使用。
▼
auth.html ファイルへフォームと javascript を実装。
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Firebase Authentication Sample</title> </head> <body style="background-color:black;color:silver"> <div id="info"></div> <form id="formLogin"> メールアドレス<input id="mail" type="mail" /> パスワード<input id="pass" type="password" autocomplete="off" /> <button id="login">ログイン</button> <button id="regist">新規登録</button> </form> <div id="formLogout"> <button id="logout">ログアウト</button> </div> <a href="./auth_.html">./auth_.html</a> <script type="module"> import { initializeApp } from 'https://www.gstatic.com/firebasejs/10.1.0/firebase-app.js'; import { getAuth , createUserWithEmailAndPassword , signInWithEmailAndPassword , onAuthStateChanged , signOut } from 'https://www.gstatic.com/firebasejs/10.1.0/firebase-auth.js'; const firebaseConfig= { apiKey: "AIzaSyAm7iF81bPgDrO9RAXC0LyJlCKX8I99999", authDomain: "myproject-99999.firebaseapp.com", projectId: "myproject-99999", storageBucket: "myproject-99999.appspot.com", messagingSenderId: "92488499999", appId: "1:92488457108:web:3e4835e5fb290378999999" }; const app= initializeApp( firebaseConfig ); const auth= getAuth(); const elementInfo= document.getElementById( "info" ); const elementMail= document.getElementById( "mail" ); const elementPass= document.getElementById( "pass" ); const elementFormLogin= document.getElementById( "formLogin" ); const elementFormLogout= document.getElementById( "formLogout" ); regist.addEventListener( "click", function( ev ){ ev.preventDefault();//イベントデフォルト動作をキャンセル let m= elementMail.value; let p= elementPass.value; createUserWithEmailAndPassword( auth, m, p ).then( rt => { console.log( "登録 OK" + rt.user.uid ); } ).catch( er => { console.log( "登録 NG" + er.code + "/" + er.message ); elementInfo.innerText= new Date().toLocaleString() + " 登録エラー"; } ); } ); login.addEventListener( "click", function( ev ){ ev.preventDefault();//イベントデフォルト動作をキャンセル let m= elementMail.value; let p= elementPass.value; signInWithEmailAndPassword( auth, m, p ).then( rt => { console.log( "ログイン OK" + rt.user.uid + "/" + rt.user.email ); console.log( rt.user ); } ).catch( er => { console.log( "ログイン NG" + er.code + "/" + er.message ); elementInfo.innerText= new Date().toLocaleString() + " ログインエラー"; } ); } ); logout.addEventListener( "click", function( ev ){ ev.preventDefault();//イベントデフォルト動作をキャンセル signOut( auth ).then( () => { console.log( "ログアウト OK" ); } ).catch( er => { console.log( "ログアウト NG" + er.code + "/" + er.message ); } ); } ); onAuthStateChanged( auth, user =>{ if( user ){ // User is signed in, see docs for a list of available properties // https://firebase.google.com/docs/reference/js/auth.user console.log( "onAuthStateChanged" + user.uid + "/" + user.email ); elementInfo.innerText= new Date().toLocaleString() + " " + user.email + " ログイン中"; elementFormLogin.style.display= "none"; elementFormLogout.style.display= "block"; }else{ console.log( "onAuthStateChanged User is signed out" ); elementInfo.innerText= new Date().toLocaleString() + " ログアウト状態"; elementFormLogin.style.display= "block"; elementFormLogout.style.display= "none"; } } ); </script> </body> </html>auth_html ファイルは認証状態を判定するだけ。
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Firebase Authentication Sample</title> </head> <body style="background-color:black;color:silver"> <div id="info"></div> <a href="./auth.html">戻る</a> <script type="module"> import { initializeApp } from 'https://www.gstatic.com/firebasejs/10.1.0/firebase-app.js'; import { getAuth , createUserWithEmailAndPassword , signInWithEmailAndPassword , onAuthStateChanged , signOut } from 'https://www.gstatic.com/firebasejs/10.1.0/firebase-auth.js'; const firebaseConfig= { apiKey: "AIzaSyAm7iF81bPgDrO9RAXC0LyJlCKX8I99999", authDomain: "myproject-99999.firebaseapp.com", projectId: "myproject-99999", storageBucket: "myproject-99999.appspot.com", messagingSenderId: "92488499999", appId: "1:92488457108:web:3e4835e5fb290378999999" }; const app= initializeApp( firebaseConfig ); const auth= getAuth(); const elementInfo= document.getElementById( "info" ); onAuthStateChanged( auth, user =>{ if( user ){ // User is signed in, see docs for a list of available properties // https://firebase.google.com/docs/reference/js/auth.user console.log( "onAuthStateChanged" + user.uid + "/" + user.email ); elementInfo.innerText= new Date().toLocaleString() + user.email + " ログイン中"; }else{ console.log( "onAuthStateChanged User is signed out" ); elementInfo.innerText= new Date().toLocaleString() + " ログアウト状態"; } } ); </script> </body> </html>■動作確認
https://remix.asia/auth.html
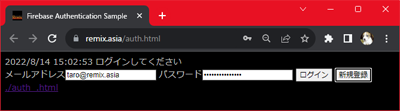
▼
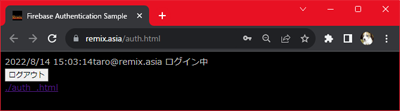
↓↑遷移してもOK
https://remix.asia/auth_.html
このままでは、auth.htm, auth_.html をダウンロード・コピー出来る為 イタズラされる恐れ。有効な https サイトからに制限する場合...▼
■Google Cloud Console
https://console.cloud.google.com/home/

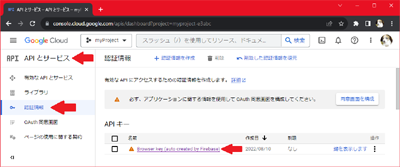
▼
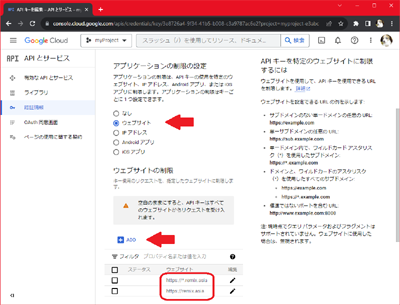
■Firebase Console でアカウント管理
https://console.firebase.google.com/

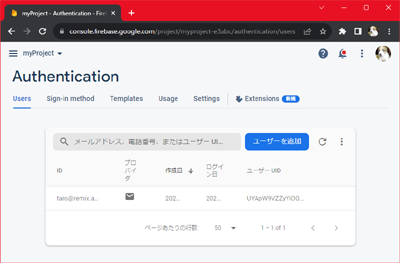